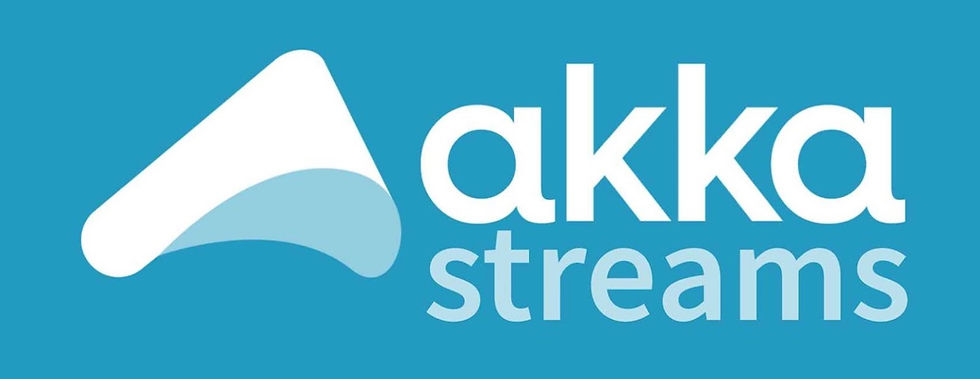
Concurrency is a critical factor in building high-performance and scalable applications. With the rise of multicore processors, it's become increasingly important to design applications leveraging concurrency to take advantage of the available processing power.
One popular toolkit for building concurrent and distributed applications in Scala is Akka. Akka is based on the Actor model, which provides a powerful abstraction for concurrent computation.
In this blog post, we'll deeply dive into Akka's Actor model and its underlying threading model, and explore how they work together to enable efficient and fault-tolerant concurrent computation.
The Actor Model in Akka
At the heart of Akka is the Actor model, which provides a high-level abstraction for concurrent computation. In the Actor model, analysis is organized around independent units of execution called actors, which communicate with each other by exchanging messages.
Each actor has its thread of execution and runs concurrently with other actors in the system. Actors are isolated from each other and communicate only through message passing, which makes them a natural fit for distributed and fault-tolerant systems.
Akka implements the Actor model using lightweight, non-blocking threads called "futures." Futures are managed by an underlying thread pool and allow multiple actors to run concurrently on a small number of threads.
Each actor has an associated mailbox that holds incoming messages until the actor is ready to process them. When the actor is ready, it dequeues and processes the message from its mailbox. Actors are designed to be lightweight and have minimal overhead, allowing many actors to be created and run concurrently.
Key features of the actor model
Below are some of the features of the actor which make them very profitable in writing code for developers:
Actors: Actors are the fundamental building blocks of an Akka system. Each actor is an independent, lightweight unit of computation that processes messages and communicates with other actors.
Message passing: In the actor model, actors communicate with each other by exchanging messages. Messages are immutable objects that can contain any type of data.
Isolation: Each actor runs in its thread and has its state, making it isolated from other actors. This helps to prevent concurrency issues such as race conditions and deadlocks.
Supervision: Akka provides a supervision hierarchy that allows actors to monitor and manage other actors. If an actor encounters an error, it can notify its supervisor, which can then take appropriate action such as restarting the actor or stopping the entire system.
Location transparency: Akka provides location transparency, which means that actors can communicate with each other regardless of their physical location. This makes it easy to build distributed systems.
Cluster support: Akka also provides cluster support, which allows actors to be distributed across multiple nodes in a cluster. This helps to improve scalability and fault tolerance.
The Threading Model in Akka
The threading model in Akka is designed to maximize concurrency and minimize contention. Akka uses a combination of event-driven and thread-based execution to achieve this goal.
At the core of Akka's threading model is a thread pool that manages a fixed number of threads. When an actor receives a message, it's placed in a message queue associated with the actor. The actor's thread is then released to process other messages or work.
When the actor is ready to process the message, it's dequeued from the message queue, and the actor's thread is blocked until the message is processed. If the actor needs to perform blocking IO, it can release its thread back to the thread pool using a Future and continue processing other messages.
In addition to the thread pool, Akka uses an event-driven model to handle I/O operations. Akka provides a set of asynchronous I/O primitives that allow actors to perform non-blocking I/O functions. When an I/O operation completes, the associated actor is notified through a callback.
The Threading Model in Akka Vs Traditional threading models
In traditional threading models, threads are managed directly by the operating system or runtime environment. Each thread is a separate unit of execution that can run concurrently with other threads. Threads share memory and state, which can lead to race conditions, deadlocks, and other concurrency issues.
In contrast, Akka's threading model is based on the actor model, which provides a higher-level abstraction for concurrency. Actors are lightweight units of computation that communicate with each other by exchanging messages. Each actor runs in its own thread, which a thread pool manages. Actors are isolated from each other and do not share states, which helps to avoid common concurrency issues.
Here are some key differences between the threading model in Akka and traditional threading models:
Actors vs. threads: In Akka, concurrency is achieved through actors, while in traditional threading models, concurrency is achieved through threads.
Message passing vs. shared memory: In Akka, actors communicate with each other by exchanging messages, while in traditional threading models, threads share memory and state.
Isolation vs. sharing: In Akka, each actor is isolated from other actors and does not share a state, which helps to prevent race conditions and other concurrency issues. In traditional threading models, threads share memory and state, which can lead to concurrency issues.
Supervision vs. manual management: Akka provides a supervision hierarchy that allows actors to monitor and manage other actors. If an actor encounters an error, its supervisor can take appropriate action. In traditional threading models, error handling and management is typically done manually.
Overall, the actor-based threading model in Akka provides a higher-level abstraction for concurrency that helps to avoid common concurrency issues. By using lightweight actors that communicate through message passing, Akka provides a scalable and fault-tolerant framework for building concurrent applications.
Akka threading model V Executor Service in Java
The Akka threading model and the ExecutorService in Java are both used to achieve concurrency, but they differ in their approach and functionality. Here are some differences between the two:
Actor-based vs Thread-based: Akka is based on the actor model of concurrency, where each actor is a lightweight unit of computation that communicates with other actors by exchanging messages. The ExecutorService in Java is based on threads, where each thread is a separate unit of computation that can be executed concurrently with other threads.
Message-passing vs Sharing Memory: In Akka, actors communicate with each other by exchanging messages, which are asynchronous and non-blocking. In the ExecutorService, threads share memory and can access the same data and resources, which can lead to concurrency issues such as race conditions and deadlocks.
Supervision vs Error Handling: In Akka, actors can be supervised by other actors, and if an actor encounters an error, its supervisor can take appropriate action such as restarting the actor or stopping the system. In the ExecutorService, error handling is typically done manually.
Scalability vs Flexibility: Akka is designed to provide a highly scalable and fault-tolerant framework for building concurrent applications, while the ExecutorService is a more general-purpose tool that can be used to execute tasks in parallel.
Features of the threading model in Akka
Akka's threading model is designed to enable efficient and scalable execution of actor-based applications. Here are some key features of Akka's threading model:
Lightweight threads: Akka uses lightweight threads, also known as "actors", to implement concurrency. These actors are isolated and do not share states, making it easier to write concurrent code without worrying about race conditions or other common concurrency issues.
Asynchronous message passing: In Akka, actors communicate with each other by sending messages. Message passing is an asynchronous operation, which means that actors do not block while waiting for messages to be processed. This helps to improve the efficiency and scalability of the system.
Non-blocking IO: Akka uses non-blocking IO operations to read and write data from external systems, such as databases or web services. This allows the system to handle a large number of concurrent connections without blocking or slowing down the system.
ThreadPool: Akka uses a thread pool to manage the execution of actors. The thread pool is configurable and can be adjusted based on the requirements of the application. This helps to ensure that the system can scale to handle large workloads.
Supervision: Akka provides a supervision hierarchy that allows actors to monitor and manage other actors. If an actor encounters an error, it can notify its supervisor, which can then take appropriate action, such as restarting the actor or stopping the entire system.
Apache Pinot and Akka
Apache Pinot is an open-source distributed columnar database designed to provide low latency, real-time analytics. Akka, on the other hand, is an open-source toolkit and runtime for building highly concurrent, distributed, and fault-tolerant systems.
While Pinot is designed to handle large volumes of data and provide fast analytical queries, Akka is designed to provide a framework for building distributed systems. As such, there are several ways in which Pinot and Akka can be used together.
One possible approach is to use Akka to manage the distributed deployment of Pinot clusters. Akka provides tools for managing distributed systems, such as cluster management, routing, and failover, which can be used to deploy and manage Pinot clusters across a distributed environment.
Another approach is to use Akka to build applications that interact with Pinot. For example, Akka actors can be used to query and retrieve data from Pinot clusters in a highly concurrent and distributed manner. Akka streams can also be used to process data streams from Pinot in real time.
To use Apache Pinot with Akka, you can start by exploring the Pinot documentation to understand its architecture, deployment, and API. You can then explore Akka's documentation to understand how to build distributed systems using its actors, streams, and other features. Finally, you can explore existing integrations or libraries that combine Akka and Pinot to help you get started quickly.
Conclusion
In this blog post, we've explored the Actor and threading models in Akka and how they work together to enable efficient and fault-tolerant concurrent computation.
Akka's Actor model provides a powerful abstraction for concurrent computation, while the threading model maximizes concurrency and minimizes contention. This combination makes Akka a natural fit for building high-performance and scalable applications.
By leveraging Akka's concurrency model, you can build applications that can handle high levels of traffic and provide a responsive user experience. With its focus on concurrency, fault tolerance, and scalability, Akka is a valuable toolkit for any developer building concurrent and distributed systems.
In summary, Akka's Actor model and threading model provide a powerful abstraction for building concurrent and distributed applications in Scala. With its lightweight threads, message-passing model, and event-driven I/O, Akka provides a flexible and efficient toolkit for building high-performance and scalable applications
Comentarios