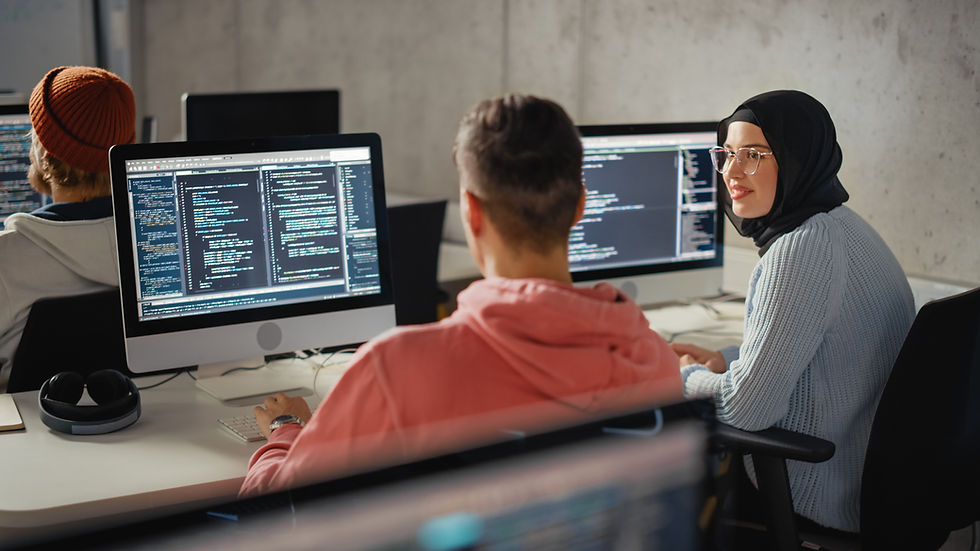
What is Akka and why is it useful?
Akka is a toolkit and runtime for building highly concurrent, distributed, and fault-tolerant systems on the JVM, written in Scala. It provides abstractions for managing concurrency, communication, and fault tolerance in distributed systems. Akka was developed by Lightbend, a company that specializes in building reactive systems. It follows an actor-based model which obeys all the principles of object-oriented programming and is built on the foundation of functional programming. Akka provides several modules that provide an abstraction like Akka HTTPS, Akka Persistence, Akka Streams, and Akka clusters which we will look at in the upcoming blogs. Akka is widely used to build microservices and for building distributed systems. It is widely used because of the following features it exhibits:
1. Scalability: Akka is designed to scale horizontally, by adding more nodes to a cluster. This makes it easy to handle large amounts of traffic and data, without having to redesign the system or add more hardware.
2. Fault tolerance: Akka is designed to handle failures gracefully, by isolating failures and recovering from them automatically. This makes it easy to build systems that are reliable and available, even in the face of failures.
3. Concurrency: Akka provides a way to manage concurrency safely and efficiently, by using actors and message passing. This makes it easy to write concurrent code that is easy to reason about and test.
4. Reactive programming: Akka is designed to support reactive programming, which is a programming paradigm that emphasizes responsiveness, resilience, and elasticity. Reactive systems are designed to handle a large volume of requests and events in a timely and efficient manner.
What are Akka Clusters?
Akka Clusters provide a way to create a group of Akka nodes that can communicate with each other and form a single logical entity. Clusters enable the creation of highly available, scalable, and fault-tolerant distributed systems.
Introduction to Akka Clusters
An Akka Cluster is a group of Akka nodes that work together to form a single logical entity. Each node in a cluster is an instance of an Akka ActorSystem, and nodes communicate with each other by exchanging messages. Nodes can be added or removed from a cluster dynamically, which allows the cluster to adapt to changes in the system.
Creating an akka cluster:
import akka.actor.ActorSystem;
import akka.cluster.Cluster;
import akka.cluster.ClusterEvent;
import akka.cluster.Member;
import akka.cluster.MemberStatus;
public class ClusterNode {
public static void main(String[] args) {
ActorSystem system = ActorSystem.create("ClusterSystem");
Cluster cluster = Cluster.get(system);
// Join the cluster
cluster.join(cluster.selfAddress());
cluster.registerOnMemberUp(() -> {
// The node is up and ready to receive messages
System.out.println("Cluster node is up and running!");
});
cluster.registerOnMemberRemoved(() -> {
// The node has been removed from the cluster
System.out.println("Cluster node has been removed!");
System.exit(0);
});
cluster.subscribe(system.actorOf(Props.create(ClusterEventListener.class)),
ClusterEvent.MemberEvent.class);
}
}
How do Akka Clusters work?
An Akka Cluster uses a peer-to-peer gossip protocol to manage communication between nodes. Nodes periodically exchange information about the state of the cluster, such as the set of nodes that are currently part of the cluster, and the state of actors that are running on each node. This information is used to maintain a consistent view of the state of the cluster across all nodes.
Akka Clusters also use a distributed pub-sub mechanism to allow nodes to publish and subscribe to messages. This mechanism enables nodes to communicate with each other in a decoupled way, which makes it easy to build loosely-coupled distributed systems.
Benefits of using Akka Clusters
Akka Clusters provide several benefits for building distributed systems:
Scalability: Akka Clusters are designed to scale horizontally, by adding more nodes to a cluster. This makes it easy to handle large amounts of traffic and data, without having to redesign the system or add more hardware.
Fault tolerance: Akka Clusters are designed to handle failures gracefully, by isolating failures and recovering from them automatically. This makes it easy to build systems that are reliable and available, even in the face of failures.
Elasticity: Akka Clusters provide a way to dynamically add or remove nodes from a cluster, which enables the system to adapt to changes in the workload or the environment.
Decoupling: Akka Clusters use a distributed pub-sub mechanism to enable nodes to communicate with each other in a decoupled way. This makes it easy to build loosely-coupled distributed systems that can be modified and extended without affecting other parts of the system.
What is Akka Persistence?
Akka Persistence is a toolkit that provides a way to store and recover the state of Akka actors. Akka Persistence enables actors to recover their state after a crash or restart, which makes it easy to build reliable and fault-tolerant systems.
Overview of Akka Persistence
Akka Persistence provides a set of APIs and tools that enable developers to implement persistence for Akka actors. Akka Persistence supports multiple persistence stores, including in-memory, JDBC, and event-sourcing databases. To define a persistent actor:
import akka.persistence.AbstractPersistentActor;
import akka.persistence.SnapshotOffer;
public class MyPersistentActor extends AbstractPersistentActor {
private int state = 0;
@Override
public String persistenceId() {
return "my-persistent-actor-id";
}
@Override
public Receive createReceiveRecover() {
return receiveBuilder()
.match(MyEvent.class, this::handleEvent)
.match(SnapshotOffer.class, this::handleSnapshotOffer)
.build();
}
@Override
public Receive createReceive() {
return receiveBuilder()
.match(MyCommand.class, this::handleCommand)
.build();
}
How Akka Persistence Works?
Akka Persistence works by storing events that represent changes in the state of actors. When an actor receives a message, it can update its state and emit an event that represents the change. The event is then stored in a persistence store.
When the actor is restarted, it can recover its state by replaying the events that were stored in the persistence store. This enables the actor to recover its state and resume processing messages from the point where it left off.
Akka Persistence also supports snapshots, which enable actors to store a snapshot of their state periodically. Snapshots can be used to speed up the recovery process, by allowing actors to recover from a snapshot instead of replaying all the events from the beginning.
Benefits of using Akka Persistence:
1. Akka Persistence provides several benefits for building reliable and fault-tolerant systems:
2. State recovery: Akka Persistence enables actors to recover their state after a crash or restart. This makes it easy to build systems that are resilient to failures, by allowing actors to resume processing messages from the point where they left off.
Persistence stores: Akka Persistence supports multiple persistence stores, including in-memory, JDBC, and event-sourcing databases. This makes it easy to choose a persistence store that meets the needs of your system.
Snapshotting: Akka Persistence supports snapshots, which enable actors to store a snapshot of their state periodically. Snapshots can be used to speed up the recovery process, by allowing actors to recover from a snapshot instead of replaying all the events from the beginning.
Event sourcing: Akka Persistence can be used with event sourcing, which provides a way to store a complete history of changes to the state of an actor. Event sourcing enables developers to build systems that are audit-ready and can be easily debugged.
How to Use Akka Persistence in an Akka Cluster?
Akka Persistence can be used in an Akka Cluster to enable actors to recover their state after a crash or restart, and to scale up or down the number of nodes in the cluster. In this section, we will explore how to use Akka Persistence in an Akka Cluster.
Setting up Akka Persistence in a Cluster
To use Akka Persistence in an Akka Cluster, you need to configure each node in the cluster to use the same persistence store. This can be done by configuring the Akka.persistence.journal.plugin and Akka.persistence.snapshot-store.plugin settings in the application.conf file.
For example, to configure Akka Persistence to use a JDBC store, you can add the following settings to the application.conf file:
akka.persistence.journal.plugin = "jdbc-journal"
akka.persistence.snapshot-store.plugin = "jdbc-snapshot-store"
You also need to configure the cluster to use the same akka.persistence.id for each actor that you want to persist. This ensures that the same actor is always recovered by the same node in the cluster.
Using Akka Persistence to Recover from Failures
One of the main benefits of using Akka Persistence in an Akka Cluster is that actors can recover their state after a crash or restart. To use Akka Persistence to recover from failures, you need to implement persistence for each actor that you want to persist.
To implement persistence for an actor, you need to extend the PersistentActor class and override the receiveCommand and receiveRecover methods. In the receiveCommand method, you handle incoming messages and update the state of the actor. In the receiveRecover method, you replay the events that were stored in the persistence store to recover the state of the actor.
Using Akka Persistence to Scale Up or Down
Another benefit of using Akka Persistence in an Akka Cluster is that it enables you to scale up or down the number of nodes in the cluster. When a new node joins the cluster, it can recover the state of actors from the persistence store, and start processing messages from the point where they left off.
To scale up or down the number of nodes in the cluster, you can use Akka Cluster Sharding, which is a built-in feature of Akka that enables actors to be distributed across multiple nodes in the cluster. Akka Cluster Sharding automatically manages the distribution of actors across the cluster and ensures that each actor is recovered by the same node that originally persisted in its state.
How to Handle Failures in an Akka Cluster?
An Akka Cluster is a distributed system, which means that it is susceptible to failures. In this section, we will explore how to handle failures in an Akka Cluster.
Understanding Failure Scenarios in an Akka Cluster
Failures can occur in an Akka Cluster in many different scenarios, such as node crashes, network partitions, and split-brain situations. When a failure occurs, the cluster must be able to recover quickly and resume normal operation.
One way to handle failures in an Akka Cluster is to use Akka Persistence and Cluster Sharding to ensure resilience.
Using Akka Persistence and Cluster Sharding for Resilience:
Akka Persistence can be used to recover actors after a failure, while Cluster Sharding can be used to distribute actors across the cluster and ensure that each actor is recovered by the same node that originally persisted in its state.
By using Akka Persistence and Cluster Sharding together, you can ensure that actors are automatically recovered after a failure and that the cluster can resume normal operation as quickly as possible.
Best Practices for Handling Failures in Akka Clusters:
In addition to using Akka Persistence and Cluster Sharding, there are several best practices that you can follow to handle failures in an Akka Cluster:
Use a Replicated Database: Use a replicated database, such as Apache Cassandra or Apache Kafka, to ensure that data is replicated across multiple nodes in the cluster. This can help prevent data loss in the event of a node failure.
Monitor Cluster Health: Monitor the health of the cluster using tools such as Akka Management or Kubernetes. This can help you identify and diagnose failures quickly.
Implement Circuit Breakers: Implement circuit breakers to prevent cascading failures in the event of a node failure. This can help ensure that the failure is contained and does not affect other nodes in the cluster.
Design for Scalability: Design your actors and messages to be scalable, so that they can handle a large number of messages and can be distributed across multiple nodes in the cluster.
Conclusion
In this blog post, we introduced the Akka toolkit and explored two of its key components, Akka Clusters, and Akka Persistence. We discussed how Akka Clusters can be used to build distributed systems that are fault-tolerant and scalable, and how Akka Persistence can be used to ensure that actors can recover their state after a failure.
If you need help with your Software engineering requirements, Please contact 'Hello@fusionpact.com'
Know more about us by visiting https://www.fusionpact.com/
Comments